Why we discarded Reactive systems architecture from our code?
This article explores our decision to move away from reactive architecture in our software project. We’ll delve into the core principles of reactive systems, the benefits of non-blocking I/O, and the challenges we faced with a reactive approach.
Understanding Reactive architecture style
Reactive encompasses a set of principles and guidelines aimed at constructing responsive distributed systems and applications, characterized by:
- Responsiveness: Capable of swiftly handling requests, even under heavy loads.
- Resilience: Able to recover from failures with minimal downtime.
- Elasticity: Can adapt to changing workloads by scaling resources accordingly.
- Message-Driven: Utilizes asynchronous messaging to enhance fault tolerance and decouple components.
One key benefit of reactive systems is their use of non-blocking I/O. This approach avoids blocking threads during I/O operations, allowing a single thread to handle multiple requests concurrently. This can significantly improve system efficiency compared to traditional blocking I/O. In traditional multithreading, blocking operations pose significant challenges in optimizing systems (Figure 1). Greedy applications consuming excessive memory are inefficient and penalize other applications, often necessitating requests for additional resources like memory, CPU, or larger virtual machines.
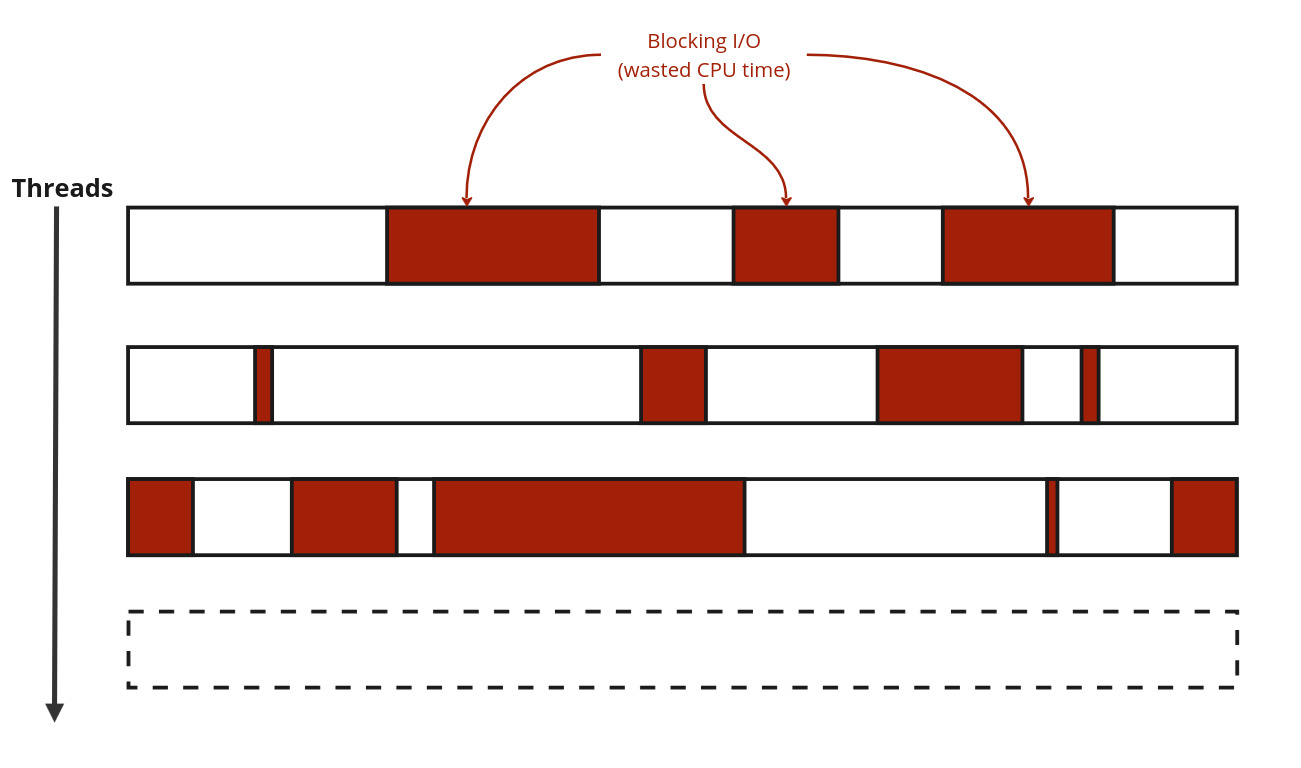
Figure 1 – Traditional Multi-threading
I/O operations are integral to modern systems, and efficiently managing them is paramount to prevent greedy behavior. Reactive systems employ non-blocking I/O, enabling a low number of OS threads to handle numerous concurrent I/O operations.
Reactive Execution Model
Although non-blocking I/O offers substantial benefits, it introduces a novel execution model distinct from traditional frameworks. Reactive programming emerged to address this issue, as it mitigates the inefficiency of platform threads idling during blocking operations (Figure 2).
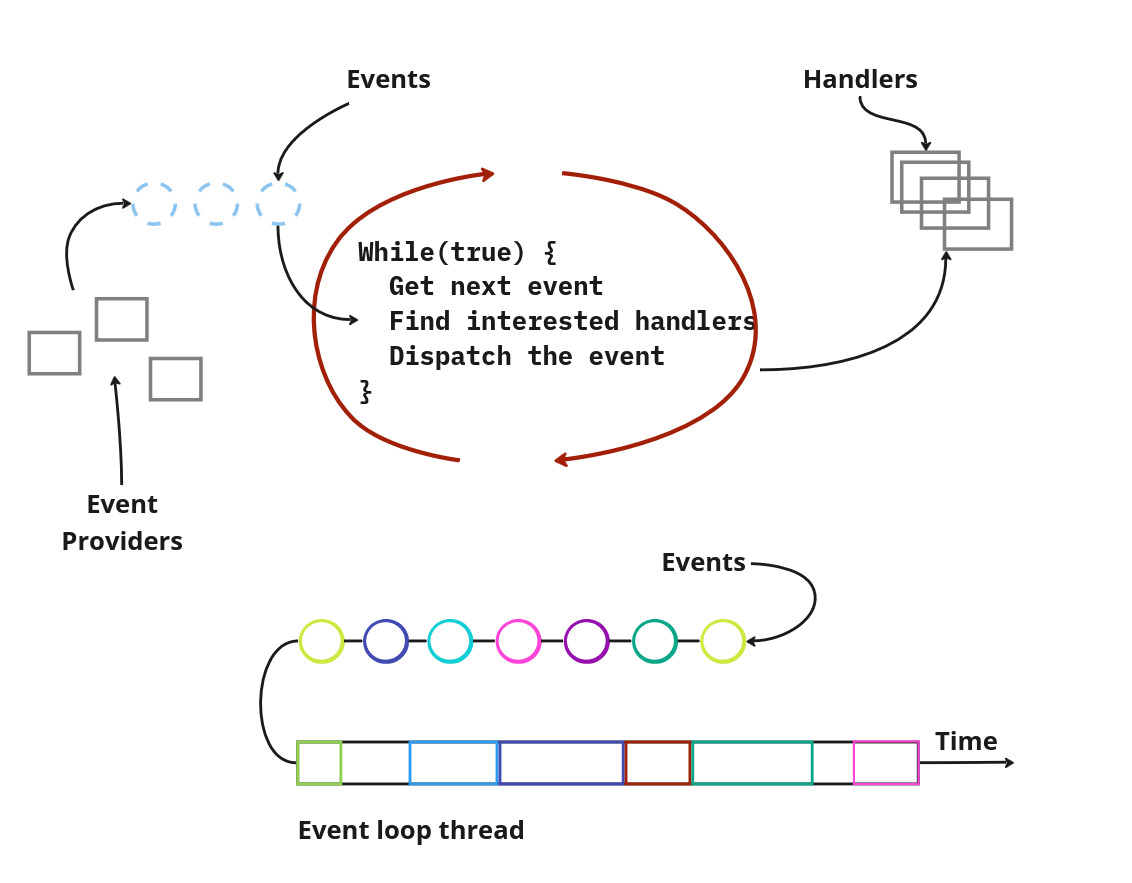
Figure 2 – Reactive Event Loop
Quarkus and Reactive
Quarkus leverages a reactive engine powered by Eclipse Vert.x and Netty, facilitating non-blocking I/O interactions. Mutiny, the preferred approach for writing reactive code with Quarkus, adopts an event-driven paradigm, wherein reactions are triggered by received events.
Mutiny offers two event-driven and lazy types:
- Uni: Emits a single event (an item or a failure), suitable for representing asynchronous actions with zero or one result.
- Multi: Emits multiple events (n items, one failure, or one completion), representing streams of items, potentially unbounded.
Challenges with Reactive
While reactive systems offer benefits, we encountered several challenges during development:
- Paradigm Shift: Reactive programming necessitates a fundamental shift in developers’ mindsets, which can be challenging, especially for developers accustomed to imperative programming. Unlike auxiliary tools like the Streams API, the reactive approach demands a complete mindset overhaul.
- Code Readability and Understanding: Reactive code poses difficulties for new developers to comprehend, leading to increased time spent deciphering and understanding it. The complexity introduced by reactive paradigms compounds this issue.
Note
“Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code. …[Therefore,] making it easy to read makes it easier to write.” ― Robert C. Martin, Clean Code: A Handbook of Agile Software Craftsmanship
- Debugging Challenges: Debugging reactive code proves nearly impossible with standard IDE debuggers due to lambdas encapsulating most code. Additionally, the loss of meaningful stack traces during exceptions further hampers debugging efforts.
- Increased Development and Testing Efforts: The inherent complexity of reactive code can lead to longer development cycles due to the time required for writing, modifying, and testing.
Here’s an example of reactive code using Mutiny to illustrate the complexity:
1Multi.createFrom().ticks().every(Duration.ofSeconds(15))
2 .onItem().invoke(() - > Multi.createFrom().iterable(configs())
3 .onItem().transform(configuration - > {
4 try {
5 return Tuple2.of(openAPIConfiguration,
6 RestClientBuilder.newBuilder()
7 .baseUrl(new URL(configuration.url()))
8 .build(MyReactiveRestClient.class)
9 .getAPIResponse());
10
11 } catch (MalformedURLException e) {
12 log.error("Unable to create url");
13
14 }
15 return null;
16}).collect().asList().toMulti().onItem().transformToMultiAndConcatenate(tuples - > {
17
18 AtomicInteger callbackCount = new AtomicInteger();
19 return Multi.createFrom().emitter(emitter - > Multi.createFrom().iterable(tuples)
20 .subscribe().with(tuple - >
21 tuple.getItem2().subscribe().with(response - > {
22 emitter.emit(callbackCount.incrementAndGet());
23
24 if (callbackCount.get() == tuples.size()) {
25 emitter.complete();
26 }
27 })
28 ));
29
30}).subscribe().with(s - > {},
31Throwable::printStackTrace, () - > doSomethingUponComplete()))
32 .subscribe().with(aLong - > log.info("Tic Tac with iteration: " + aLong));
Future Outlook-Project Loom and Beyond
Project Loom, a recent development in the Java ecosystem, promises to mitigate the issues associated with blocking operations. By enabling the creation of thousands of virtual threads without hardware changes, Project Loom could potentially eliminate the need for a reactive approach in many cases.
Note
“Project Loom is going to kill Reactive Programming” - Brian Goetz
Conclusion
In conclusion, our decision to move away from reactive architecture style a pragmatic approach to our project’s long-term maintainability. While reactive systems offer potential benefits, the challenges they presented for our team outweighed those advantages in our specific context.
Importantly, this shift did not compromise performance. This is a positive outcome, as it demonstrates that a well-designed non-reactive(imperative) architecture can deliver the necessary performance without the complexity associated with reactive architecture in our case.
As we look towards the future, the focus remains on building a codebase that is not only functional but also easy to understand and maintain for developers of all experience levels. This not only reduces development time but also fosters better collaboration and knowledge sharing within the team.
In the graph below, the X-axis represents the increasing complexity of our codebase as it evolves, while the Y-axis depicts the time required for these developmental changes.
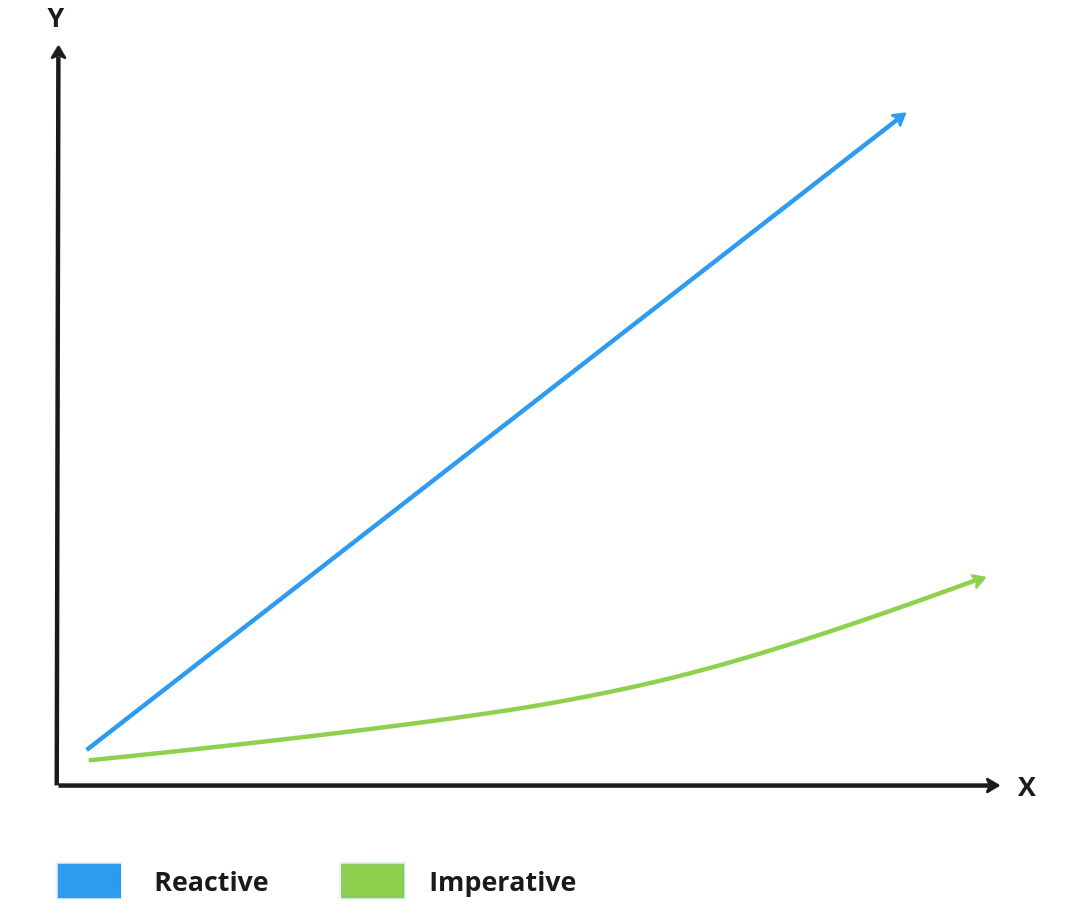