The Challenge of Evolving Schemas in Message Queues
In systems built on message queues like RabbitMQ or Pub/Sub, schema evolution is inevitable. It allows you to adapt to changing needs and add new features, but it can also introduce challenges. When updating schemas, ensuring a smooth transition is crucial to avoid disrupting existing processes and consumers. Let’s explore a scenario where your schema undergoes significant changes:
Initial Payload (Version 1.0):
1{
2 "eventType": "user_created",
3 "version": "1.0",
4 "timestamp": "2024-01-15T08:00:00Z",
5 "data": {
6 "id": "123456789",
7 "username": "john_doe",
8 "name": "John Doe",
9 "email": "john.doe@example.com",
10 "age": 30,
11 "gender": "male",
12 "address": {
13 "street": "123 Main St",
14 "city": "Anytown",
15 "state": "CA",
16 "postal_code": "12345",
17 "country": "USA"
18 },
19 "phone_numbers": [
20 {
21 "type": "home",
22 "number": "123-456-7890"
23 }
24 ],
25 "is_active": true,
26 "registration_date": "2024-01-15T08:00:00Z"
27 }
28}
Then, suppose you introduce changes to this JSON structure as follows(Version 2.0):
1{
2 "eventType": "user_created",
3 "version": "2.0",
4 "timestamp": "2024-01-15T08:00:00Z",
5 "data": {
6 "userId": "123456789",
7 "profileDetails": {
8 "username": "john_doe",
9 "fullName": "John Doe",
10 "email": "john.doe@example.com",
11 "age": 30,
12 "gender": "male"
13 },
14 "contact": {
15 "address": {
16 "street": "123 Main St",
17 "city": "Anytown",
18 "state": "CA",
19 "postalCode": "12345",
20 "country": "USA"
21 },
22 "phoneNumbers": [
23 {
24 "type": "home",
25 "number": "123-456-7890"
26 }
27 ]
28 },
29 "isActive": true,
30 "createdAt": "2024-01-15T08:00:00Z"
31 }
32}
Now, you need to migrate your system to handle these changes seamlessly.
A Solution
-
Create a New Topic: Establish a new topic dedicated to publishing messages adhering to the updated schema versions. Producer publishes messages to the appropriate topic based on the schema used. This allows gradual migration and facilitates parallel operation of old and new consumers.
-
Update the Producer: Modify your producer to generate messages in the new schema format and direct these messages to the newly created topic.
-
Implement the Adapter Pattern: Develop an adapter component responsible for listening to messages in the new topic. The adapter, following the adapter pattern, translates messages from the new format to the old format and then transmits them to the original topic.
-
Migration of Consumers: Gradually migrate your consumers by redirecting them to subscribe to the new topic instead of the old one.
-
Decommission the Adapter: Once all consumers have successfully migrated, decommission the adapter, and remove the old topic from the system.
Implementation Procedure
- User creation event version 1.
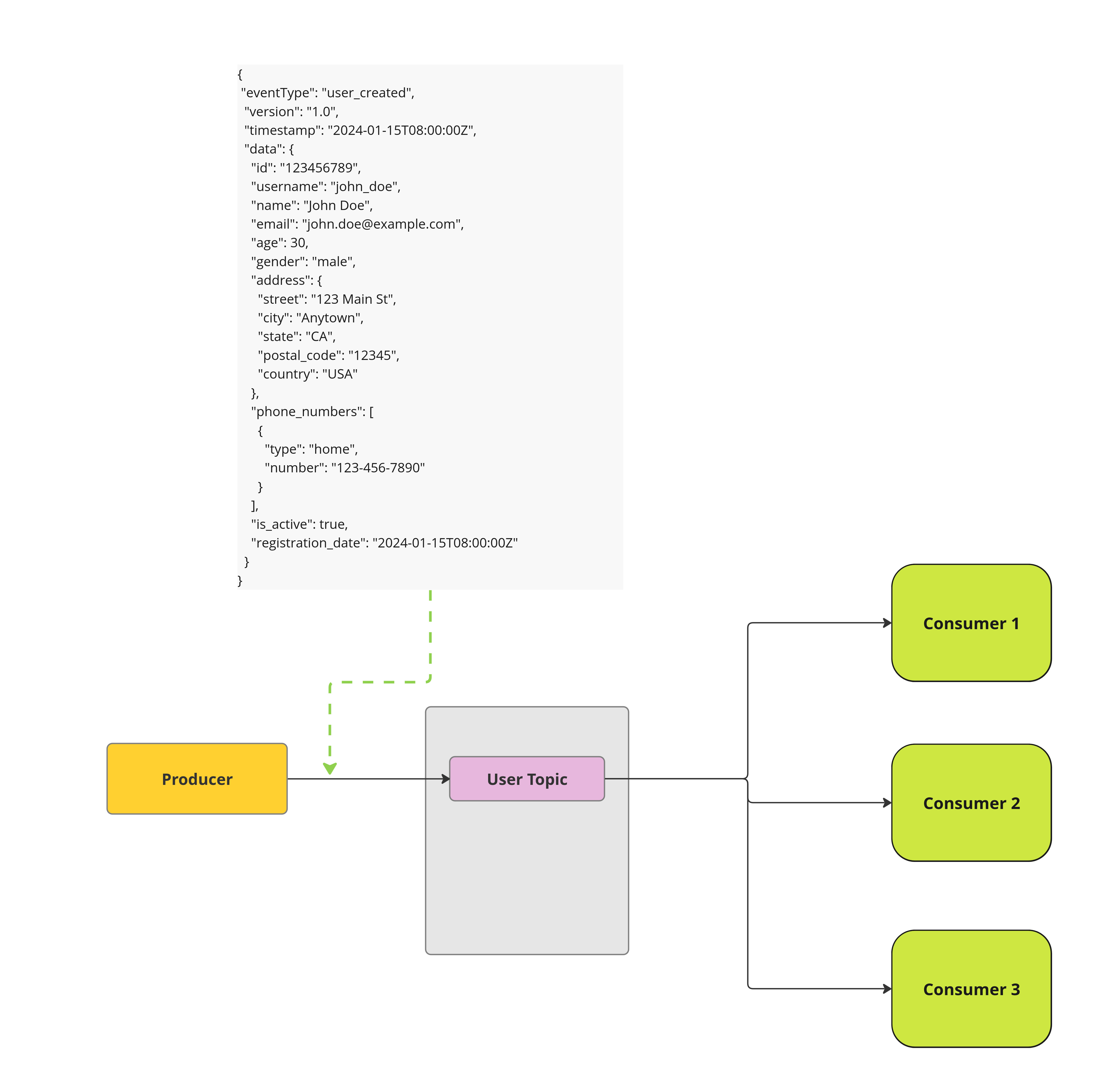
- The adapter listens for messages in the new topic, translates them to the old format, and then forwards them to the old topic
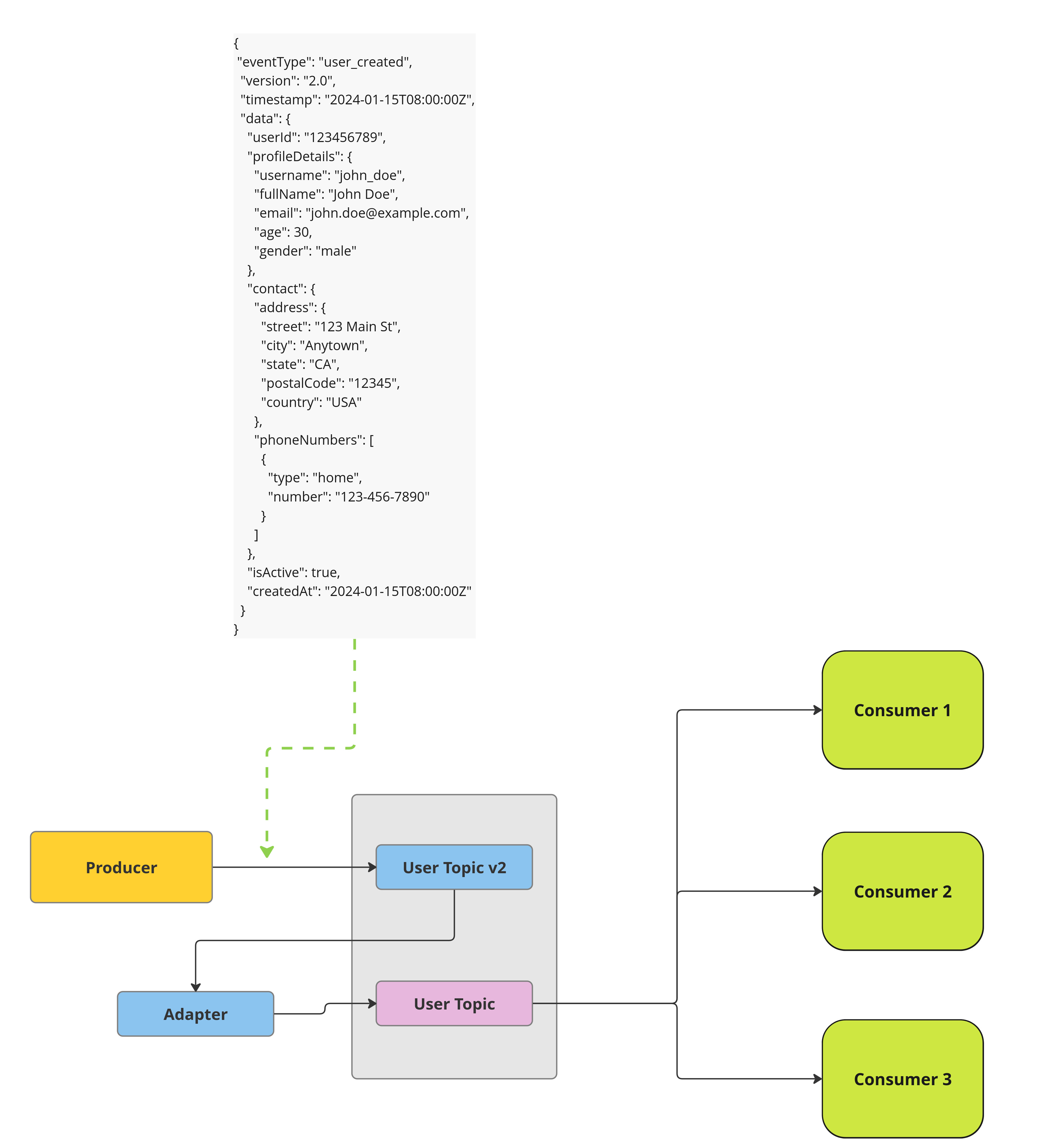
- In the next step, one consumer has migrated, with the other soon to follow.
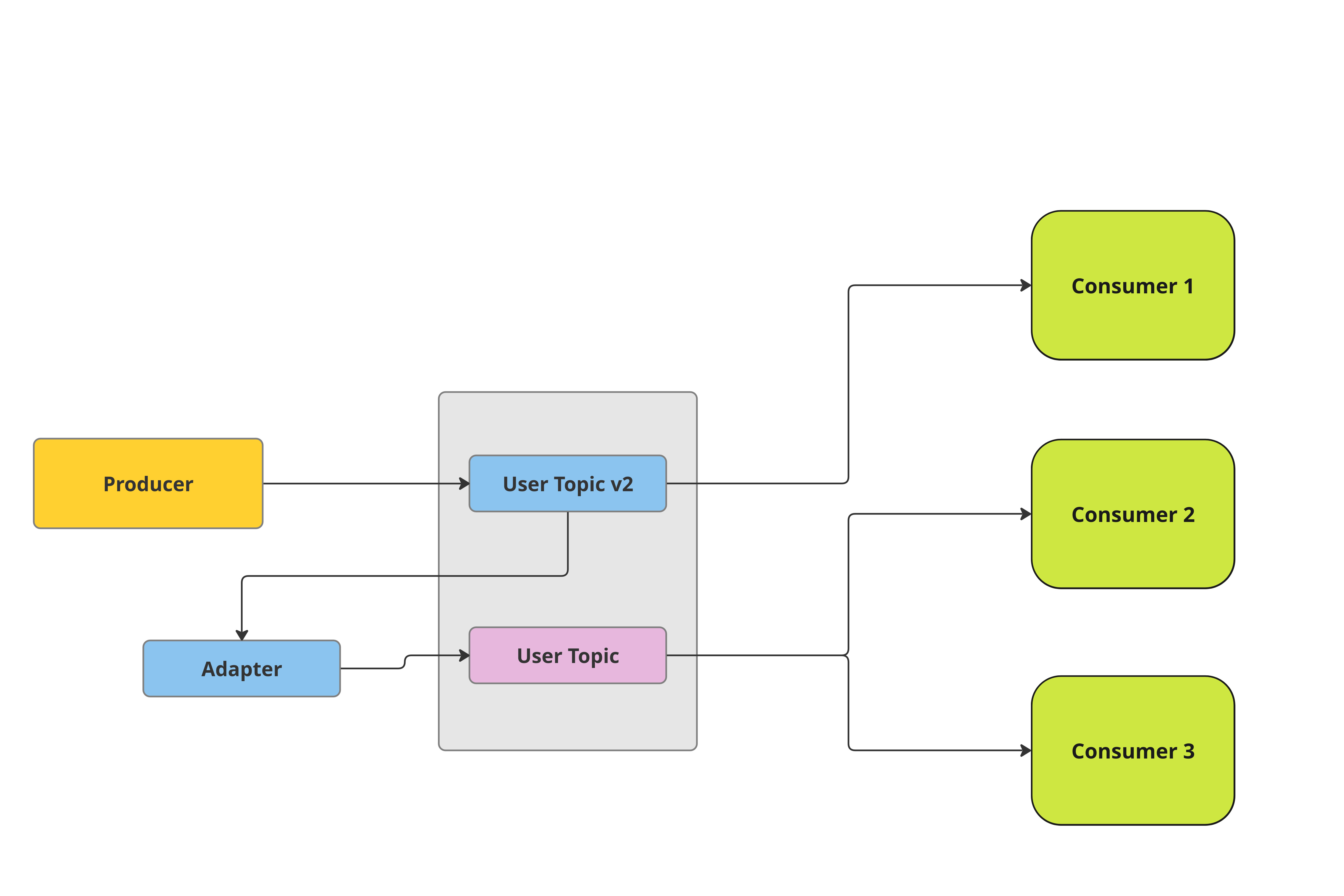
- In the final step, the adapter becomes obsolete, and the old topic is no longer needed.
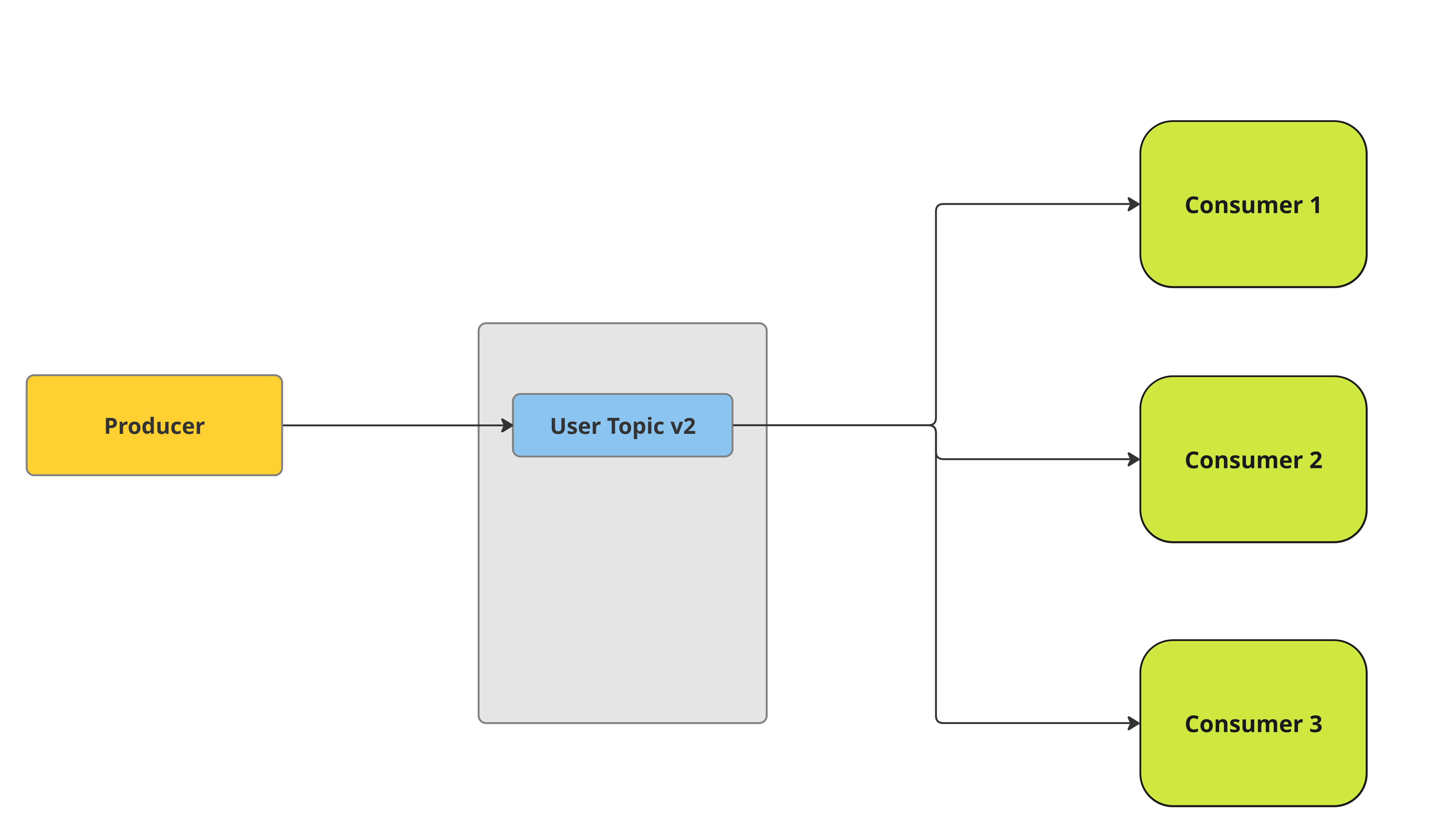
Benefits of this approach
-
Backward Compatibility: The Adapter ensures backward compatibility by acting as a bridge between the old and new schema formats, allowing existing Consumers to continue processing messages without disruption.
-
Flexibility: Leveraging the adapter pattern provides flexibility in accommodating changes in schema versions or message formats, enabling incremental updates without impacting existing functionality.
-
Risk Mitigation: The adapter pattern serves as a risk mitigation strategy by isolating the impact of schema changes, reducing the risk of disruptions or errors in message processing.
-
Clear Versioning: Versioned topics and a schema registry provide clarity and transparency, making debugging and monitoring easier.
Conclusion
Navigating schema evolution in message queue systems demands a strategic approach to ensure seamless transitions. By adopting the outlined solution, organizations can effectively address the challenges associated with schema updates while preserving operational continuity.
The proposed strategy acknowledges the inevitability of schema evolution and provides a structured approach to handle changes efficiently. By leveraging the Adapter Pattern, organizations can bridge the gap between old and new schema formats, facilitating backward compatibility and minimizing disruptions to existing processes.
Furthermore, the approach offers flexibility and risk mitigation, allowing for incremental updates and isolating the impact of schema changes. This ensures that system evolution occurs smoothly, with minimal risk to operational stability.