Exploring Pinning in JVM's Virtual Thread Mechanism
Java’s virtual threads offer a lightweight alternative to traditional OS threads, enabling efficient concurrency management. But understanding their behavior is crucial for optimal performance. This blog post dives into pinning, a scenario that can impact virtual thread execution, and explores techniques to monitor and address it.
Virtual Threads: A Lightweight Concurrency Approach
Java’s virtual threads are managed entities that run on top of the underlying operating system threads (carrier threads). They provide a more efficient way to handle concurrency compared to creating numerous OS threads, as they incur lower overhead. The JVM maps virtual threads to carrier threads dynamically, allowing for better resource utilization.
-
Managed by the JVM: Unlike OS threads that are directly managed by the operating system, virtual threads are created and scheduled by the Java Virtual Machine (JVM). This allows for finer-grained control and optimization within the JVM environment.
-
Reduced Overhead: Creating and managing virtual threads incurs significantly lower overhead compared to OS threads. This is because the JVM can manage a larger pool of virtual threads efficiently, utilizing a smaller number of underlying OS threads.
-
Compatibility with Existing Code: Virtual threads are designed to be seamlessly integrated with existing Java code. They can be used alongside traditional OS threads and work within the familiar constructs like Executor and ExecutorService for managing concurrent.
The figure below shows the relationship between virtual threads and platform threads:
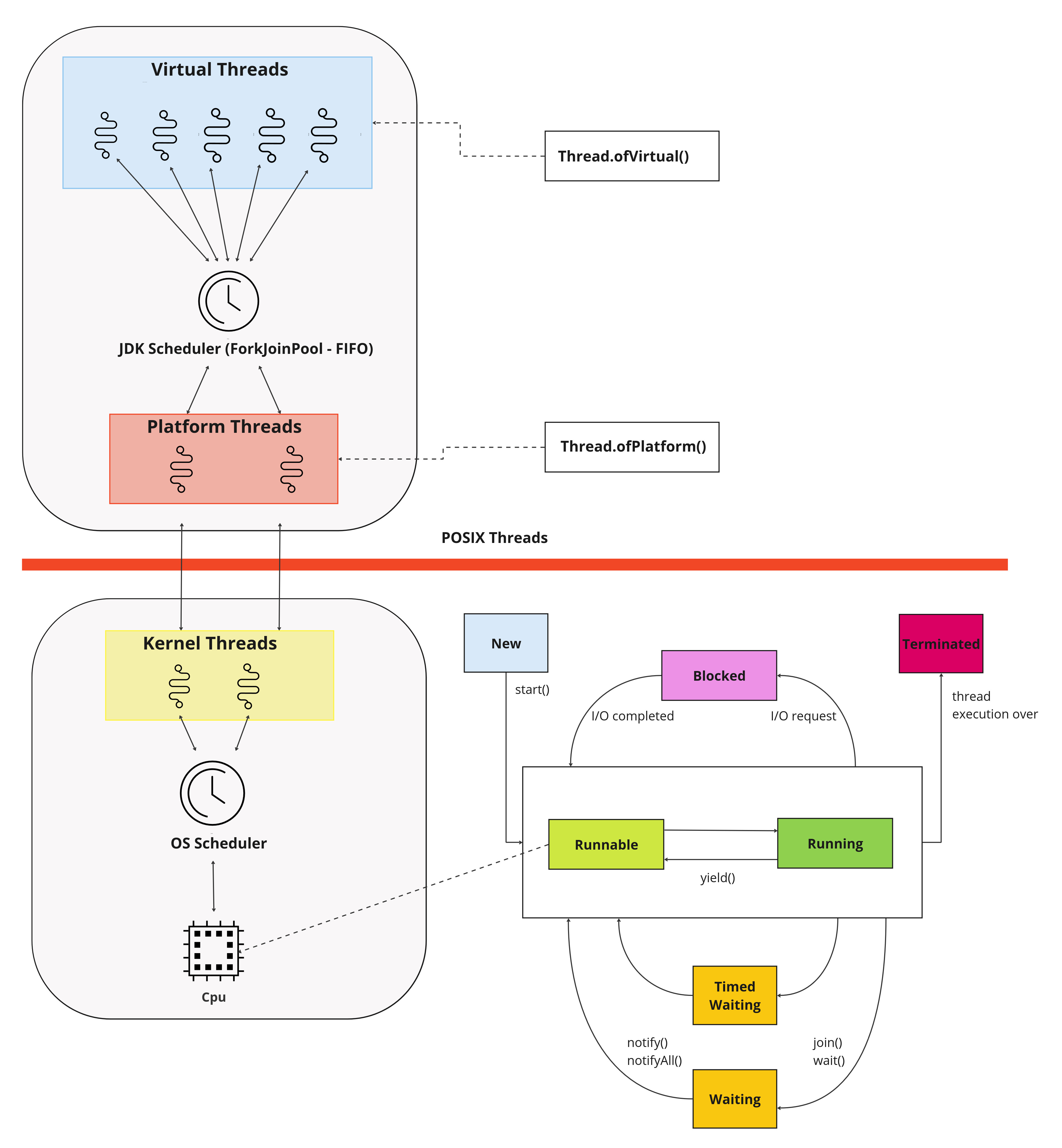
Pinning: When a Virtual Thread Gets Stuck
Pinning occurs when a virtual thread becomes tied to its carrier thread. This essentially means the virtual thread cannot be preempted (switched to another carrier thread) while it’s in a pinned state. Here are common scenarios that trigger pinning:
- Synchronized Blocks and Methods: Executing code within a synchronized block or method leads to pinning. This ensures exclusive access to shared resources, preventing data corruption issues.
Code Example:
1import java.util.concurrent.ExecutorService;
2import java.util.concurrent.Executors;
3
4public class Main {
5
6 public static void main(String[] args) throws InterruptedException {
7
8 final Counter counter = new Counter();
9
10 Runnable task = () -> {
11 for (int i = 0; i < 1000; i++) {
12 counter.increment();
13 }
14 };
15
16 Thread thread1 = new Thread(task);
17 Thread thread2 = new Thread(task);
18
19 thread1.start();
20 thread2.start();
21
22 thread1.join();
23 thread2.join();
24
25 System.out.println("Final counter value: " + counter.getCount());
26 }
27}
28
29class Counter {
30
31 private int count = 0;
32
33 public synchronized void increment() {
34 count++;
35 }
36
37 public synchronized int getCount() {
38 return count;
39 }
40}
In this example, when a virtual thread enters the synchronized
block, it becomes pinned to its
carrier thread, but this is not always true.
Java’s synchronized
keyword alone is not enough to cause thread pinning in virtual
threads. For thread pinning to occur, there must be a blocking point within a synchronized
block
that causes a virtual thread to trigger park, and ultimately disallows unmounting from its carrier
thread. Thread pinning could cause a decrease in performance as it would negate the benefits of
using lightweight/virtual threads.
Whenever a virtual thread encounters a blocking point, its state is transitioned to PARKING
.
This state transition is indicated by invoking the VirtualThread.park()
method:
1// JDK core code
2void park() {
3 assert Thread.currentThread() == this;
4 // complete immediately if parking permit available or interrupted
5 if (getAndSetParkPermit(false) || interrupted)
6 return;
7 // park the thread
8 setState(PARKING);
9 try {
10 if (!yieldContinuation()) {
11 // park on the carrier thread when pinned
12 parkOnCarrierThread(false, 0);
13 }
14 } finally {
15 assert (Thread.currentThread() == this) && (state() == RUNNING);
16 }
17}
Let’s take a look at a code sample to illustrate this concept:
1import java.util.concurrent.ExecutorService;
2import java.util.concurrent.Executors;
3
4public class Main {
5
6 public static void main(String[] args) {
7
8 Counter counter = new Counter();
9
10 Runnable task = () -> {
11 for (int i = 0; i < 100; i++) {
12 counter.increment();
13 }
14 };
15
16 Thread thread1 = Thread.ofVirtual().start(task);
17 Thread thread2 = Thread.ofVirtual().start(task);
18
19 try {
20 thread1.join();
21 thread2.join();
22 } catch (InterruptedException e) {
23 throw new RuntimeException(e);
24 }
25
26 System.out.println("Final counter value: " + counter.getCount());
27 }
28}
29
30class Counter {
31
32 private int count = 0;
33
34 public void increment() {
35 synchronized (this) {
36 try {
37 Thread.sleep(100); // This simulates a blocking call within the synchronized block
38 } catch (InterruptedException e) {
39 e.printStackTrace();
40 }
41 count++;
42 }
43 }
44
45 public synchronized int getCount() {
46 return count;
47 }
48}
- Native Methods/Foreign Functions: Running native methods or foreign functions can also cause pinning. The JVM might not be able to efficiently manage the virtual thread’s state during these operations.
Monitoring Pinning with -Djdk.tracePinnedThreads=full
The -Djdk.tracePinnedThreads=full
flag is a JVM startup argument that provides detailed tracing
information about virtual thread pinning. When enabled, it logs events like:
- Virtual thread ID involved in pinning
- Carrier thread ID to which the virtual thread is pinned
- Stack trace indicating the code section causing pinning
Note
Use this flag judiciously during debugging sessions only, as it introduces performance overhead.
- Compile the our demo code:
1javac Main.java
- Star the compiled code with the
-Djdk.tracePinnedThreads=full
flag:
1java -Djdk.tracePinnedThreads=full Main
- Observe the output in the console, which shows detailed information about virtual thread pinning.:
1Thread[#29,ForkJoinPool-1-worker-1,5,CarrierThreads]
2java.base/java.lang.VirtualThread$VThreadContinuation.onPinned(VirtualThread.java:183)
3java.base/jdk.internal.vm.Continuation.onPinned0(Continuation.java:393)
4java.base/java.lang.VirtualThread.parkNanos(VirtualThread.java:621)
5java.base/java.lang.VirtualThread.sleepNanos(VirtualThread.java:791)
6java.base/java.lang.Thread.sleep(Thread.java:507)
7Counter.increment(Main.java:38) <== monitors:1
8Main.lambda$main$0(Main.java:13)
9java.base/java.lang.VirtualThread.run(VirtualThread.java:309)
10
11Final counter value: 200
Fixing Pinning with Reentrant Locks
Pinning is an undesirable scenario which impedes the performance of virtual threads. Reentrant locks serve as an effective tool to counteract pinning.
Here’s how you can use Reentrant
locks to mitigate pinning situations:
1import java.util.concurrent.ExecutorService;
2import java.util.concurrent.Executors;
3import java.util.concurrent.locks.ReentrantLock;
4
5public class Main {
6
7 public static void main(String[] args) {
8
9 Counter counter = new Counter();
10
11 Runnable task = () -> {
12 for (int i = 0; i < 100; i++) {
13 counter.increment();
14 }
15 };
16
17 Thread thread1 = Thread.ofVirtual().start(task);
18 Thread thread2 = Thread.ofVirtual().start(task);
19
20 try {
21 thread1.join();
22 thread2.join();
23 } catch (InterruptedException e) {
24 throw new RuntimeException(e);
25 }
26
27 System.out.println("Final counter value: " + counter.getCount());
28 }
29}
30
31class Counter {
32
33 private int count = 0;
34 private final ReentrantLock lock = new ReentrantLock();
35
36 public void increment() {
37 lock.lock();
38 try {
39 Thread.sleep(100); // This simulates a blocking call
40 count++;
41 } catch (InterruptedException e) {
42 e.printStackTrace();
43 } finally {
44 lock.unlock();
45 }
46 }
47
48 public int getCount() {
49 return count;
50 }
51}
In the updated example, we use a ReentrantLock
instead of a synchronized
block. The thread can
acquire the lock and release it immediately after it completes its operation,
potentially reducing the duration of pinning compared to a synchronized
block which might hold the
lock for a longer period.
In Conclusion
Java’s virtual threads stand as a testimony to the evolution and the capabilities of the language. They offer a fresh, lightweight alternative to traditional OS threads, providing a bridge to efficient concurrency management. Taking the time to dig deep and understand key concepts such as thread pinning can equip developers with the know-how to leverage the full potential of these lightweight threads. This knowledge not only prepares developers for leveraging upcoming features but also empowers them to resolve complex concurrency control issues more effectively in their current projects.